JavaScript check if key exists — it’s a fundamental skill every developer needs to master.
In this guide, I’ll walk you through 5 battle-tested ways to check if a key exists in JavaScript objects. We’ll talk pros, cons, and when each method makes sense.
By the end of this article, you’ll know which method to use in any situation – whether you’re working with nested objects, dealing with inherited properties, or need the straightforward solution that works.
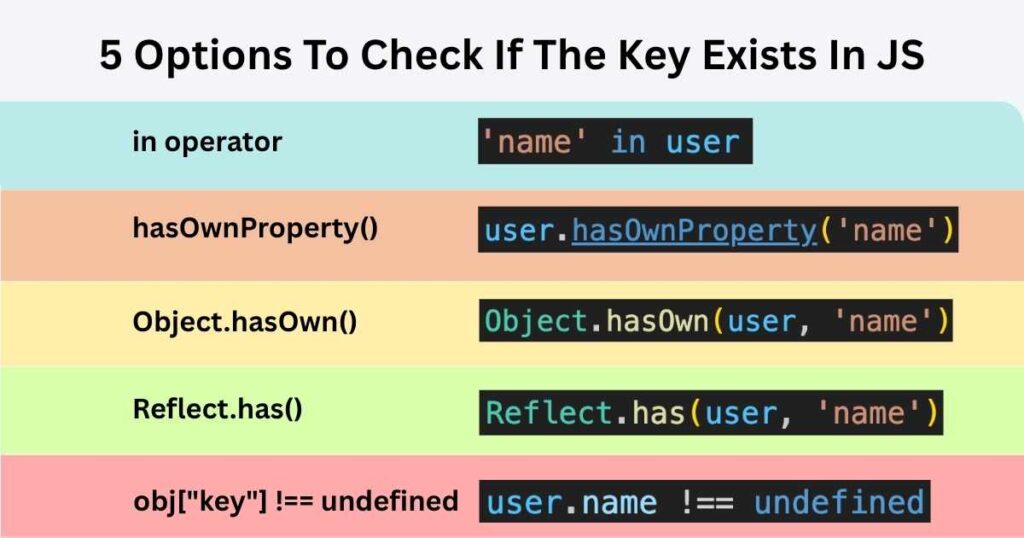
What Is The Best Way Javascript Check If Key Exists?
Checking if a key exists in a JavaScript object is something I do almost every day.
We’ve got JS objects and you wanna make sure a key is there before you try to use it. That’s where this “check if key exists” idea comes in handy.
There’s no single “best” method that works for every situation. The in operator is probably the easiest way to check for keys. It tells whether the key exists in the object or its prototype chain.
Many developers also use the hasOwnProperty() method. It only checks for keys that belong directly to the object and won’t look at inherited properties.
The newer Object.hasOwn() method is becoming more popular. It works like hasOwnProperty but is more reliable.
In my experience teaching JavaScript, the in operator works best for most situations. It’s simple to use and easy to understand. But if you’re worried about inherited properties, go with hasOwnProperty or Object.hasOwn.
1. Using in
Operator to Check Key in Object — Popular JavaScript Check If Key Exists
The in
operator is one of the simplest ways to check if a key exists in a JavaScript object. It’s got a super simple vibe and returns true
for inherited stuff.
const user = { name: 'Eugene' }; console.log('name' in user); // true console.log('toString' in user); // true — inherited from Object.prototype! console.log('email' in user); // false — key doesn't exist
The syntax is super straightforward — just put the key name (as a string) on the left side of the in
keyword and the object on the right. If the key exists, you’ll get true
. If not, you’ll get false
.
You can work with all data types, including Symbols. It also works with arrays, where the indexes are the keys.
The in
operator checks the entire prototype chain even if the inherited key comes from an object’s prototype. It walks the chain, and that’s the whole point!
That’s super useful working with objects from a library, or passed in from somewhere like an API endpoint. Learn more about “What Is an API Call? Everything You Need to Know in 5 Minutes“!
You don’t always know what’s inherited and not — and sometimes you don’t even care.
✅ Why Use in
?
- Straightforward syntax — just one keyword
- Checks all keys: own + inherited
- Supports symbols and strings
- Looks like natural language
However, that also means you might think a key exists, but it’s actually inherited, not something you set directly. If you’re not careful, you’ll think your object has a key — but it’s really coming from Object.prototype.
The in
operator has excellent browser support and is generally fast for most use cases. However, for performance-critical code, hasOwnProperty()
is slightly faster since it doesn’t traverse the prototype chain.
In TypeScript, in
works as a type guard. It narrows down unions and helps the compiler figure out types.
interface Dog { bark: () => void } interface Cat { meow: () => void } function speak(animal: Dog | Cat) { if ('bark' in animal) { animal.bark(); } else { animal.meow(); } }
Learn more about TS in my “What Is TypeScript: 5 Features That Make JavaScript Better” post.
Anyway — bottom line — the in
operator is short, quick, and walks the prototype chain. If you’re fine with inherited keys, it’s your best bet. If you’re only interested in direct properties, check out hasOwnProperty()
or Object.hasOwn()
instead.
2. Using hasOwnProperty()
Method to Detect Key
The hasOwnProperty()
method only looks for properties that belong directly to the JavaScript object itself, NOT the inherited props.
Using hasOwnProperty() is more precise than the in operator as it only checks for direct properties.
const user = { name: "Eugene", age: 30 }; if (user.hasOwnProperty("name")) { console.log("Name exists!"); // This will run }
This code creates a user object with name
and age
. Then it checks if “name” exists as a direct property. Since it does, the message prints out.
This method returns true or false. It returns true when the object has the property as its own. It returns false if the property doesn’t exist or is inherited.
const obj = { name: 'John' }; console.log(obj.hasOwnProperty('name')); // true console.log(obj.hasOwnProperty('toString')); // false (inherited from prototype)
This example shows how it ignores inherited properties like “toString”, which all JS objects get from Object.prototype.
hasOwnProperty()
has some downsides too. It can be overridden.
For safety, some pros use this pattern instead:
Object.prototype.hasOwnProperty.call(obj, 'propertyName');
This code calls the hasOwnProperty method indirectly. This prevents problems if someone has messed with the original method. It’s a bit clunky but SUPER safe!
TypeScript users get extra benefits as it provides some extra safety nets.
TypeScript’s type checking sometimes makes runtime checks unnecessary. But when you need to, hasOwnProperty() still comes in handy:
interface User { name: string; age?: number; // Optional property } function processUser(user: User) { // TypeScript ensures name exists, but age might not if (user.hasOwnProperty('age')) { console.log(user.age); } }
In short, with
Javascript check if a key exists directly on an object while avoiding prototype chain confusion. Modern JavaScript introduced Object.hasOwn() as a more concise alternative. But hasOwnProperty() remains widely used and understood!hasOwnProperty()
3. Using Object.hasOwn() for Safe JavaScript Check If Key Exists
Object.hasOwn() is the new kid on the block — and it’s awesome, if you ask me! This method came with ES2022 and makes our lives easier when javascript check if key exists.
const user = { name: "John", age: 30 }; if (Object.hasOwn(user, "name")) { console.log("Name exists!"); }
This code checks if the user object has a “name” property. Since it does, the message "Name exists!"
will show in the console.
A lot of folks now recommend using Object.hasOwn() instead of the old .hasOwnProperty() method. It works better when an object doesn’t have its usual functions.
Object.hasOwn() works with objects created using Object.create(null). The hasOwnProperty() method would fail with these objects.
It also works when someone messes with the hasOwnProperty() method. Look at this example:
const weirdObj = { hasOwnProperty: function() { return false; }, key: "value" }; console.log(weirdObj.hasOwnProperty("key")); // Returns false (wrong!) console.log(Object.hasOwn(weirdObj, "key")); // Returns true (correct!)
Here, I changed how hasOwnProperty works. Now it always returns false. But Object.hasOwn() still works right and tells us the key exists.
Object.hasOwn() works in newer browsers — Chrome 93+, Firefox 92+, Safari 15.4+. But older browsers (lookin’ at you, Internet Explorer) don’t support it
And, one more example in TypeScript:
interface Product { id: number; name: string; price?: number; } function processProduct(product: Product) { if (Object.hasOwn(product, 'price')) { return product.price * 0.9; } return 'Price not available'; }
In this snippet, we’re checking if the product has a price. If it does, we give it a 10% discount. If not, we just return a message instead.
Most devs I know love how Object.hasOwn() just works. If you want a better way to check keys — use Object.hasOwn() when you can.
4. Using Reflect.has() for Key Lookup
Reflect.has() is part of a bigger set of methods called the Reflect API. If you’re building complex apps with JS Proxies, Reflect.has() is a tool worth knowing. For simple key checks, the in
operator works just fine.
Reflect.has() is a function that tells if an object has a specific property. It works just like the in
operator but comes in a function form. It checks both the object’s own properties AND inherited ones.
const user = { name: "John", age: 30 }; // Using Reflect.has() to check if key exists if (Reflect.has(user, "name")) { console.log("Name property exists!"); } console.log(Reflect.has(user, "toString")); // true
Provide the object you want to check with the property name you’re looking for, and Reflect.has() gives back true or false
Reflect.has() really shines when working with Proxies. These are special objects that can intercept operations. It also feels more consistent when you’re already using other Reflect methods in your code. Reflect.
It’s great when you’re doing meta-programming — code that works with other code. Outside meta-programming, it looks strange to team members unfamiliar with Reflect.
5. Checking obj[“key”] !== undefined (May Fail with Undefined Values)
Checking if a property is undefined is one of the most common ways devs in JS check if key exists. It’s simple and straightforward, but has some tricky problems.
const car = { make: 'Toyota', model: 'Corolla' }; console.log(car.make !== undefined); // true console.log(car.year !== undefined); // false
This code creates a car
object with make
and model
and checks if car.make
and car.year
aren’t undefined
.
The approach is simple enough — just check if something isn’t undefined. If it returns true, the property exists. If false, it probably doesn’t exist.
The big problem is that this method can’t distinguish between missing keys and undefined values.
const person = { name: 'John', age: undefined }; console.log(person.name !== undefined); // true console.log(person.age !== undefined); // false console.log(person.address !== undefined); // false
This example shows the trap. We have a person
object where the age
property exists but is set to undefined
. The code checks three properties – name (which exists), age (which exists but is undefined), and address (which doesn’t exist at all). Both age and address checks return false, but for totally different reasons!
Checking for undefined-ness is not an accurate way of testing whether a key exists. What if the key exists but the value is actually undefined?
I’ve seen this issue pop up in real projects, especially when working with API endpoints that return undefined values. A safer approach using Object.hasOwn(), hasOwnProperty(), or ‘in‘ operator.
If you’re using TypeScript, the type system helps catch these issues:
interface User { name: string; email?: string | undefined; // Might be undefined } function processUser(user: User) { // TypeScript knows email might be undefined if (user.email !== undefined) { sendEmail(user.email); } }
TS knows “email” might be undefined — and helps you avoid mistakes.
While obj[“key”] !== undefined is tempting because of its simplicity, it’s not the most reliable method. For critical code, use the in
operator or hasOwnProperty()
instead.
Unreliable Truthy Check With if (obj.key) — NOT RECOMMENDED!
A truthy check if (obj.key)
is one of the simplest approaches — but it’s also one of the most problematic! While simple, it can’t tell the difference between falsy and non-existent values.
const car = { make: 'Toyota', model: 'Corolla', electric: false }; if (car.make) { console.log('make exists and is truthy'); } // Works as expected if (car.electric) { console.log('This will not run even though the property exists'); } // Fails because the value is falsy
Here, car.electric
exists, but the code acts like it doesn’t. That’s because its value is false
, which is falsy. So the check fails even though the key exists!
I’ve been bitten by truthy checks so often that I avoid them completely now. Another common issue happens with numbers that are zero:
const user = { name: "John", points: 0 }; if (user.points) { console.log(`User has ${user.points} points`); } else { console.log("No points found"); }
This will say “No points found” even though the user has a points property (it’s just set to zero)!
Remember that null, undefined, false, 0, NaN, and empty string are FALSY, everything else is truth!
DON’T use truthy checks to see if keys exist!! Use proper methods (hasOwnProperty or Object.hasOwn()) that actually check for existence.
Conclusion — JavaScript Check If Key Exists
JavaScript check if key exists methods give you several options for different situations.
The in operator works great for most cases, while hasOwnProperty() and Object.hasOwn() help when you need to avoid inherited properties.
The best method depends on your specific needs. Take some time to understand each approach rather than memorizing just one way!