Not all code is equal! Among the various types, “spaghetti code” is a real bad guy!
Like a bowl of spaghetti of intertwined noodles, spaghetti code features a complex (knotted) structure that makes understanding and modifying it challenging.
This type of code is poorly structured and difficult to read, understand, and, most importantly, maintain!
My post defines spaghetti code, illustrates some basic examples, and provides my insights on how to prevent it.
What is Spaghetti Code?
The term “spaghetti” describes a particularly tangled and messy situation.
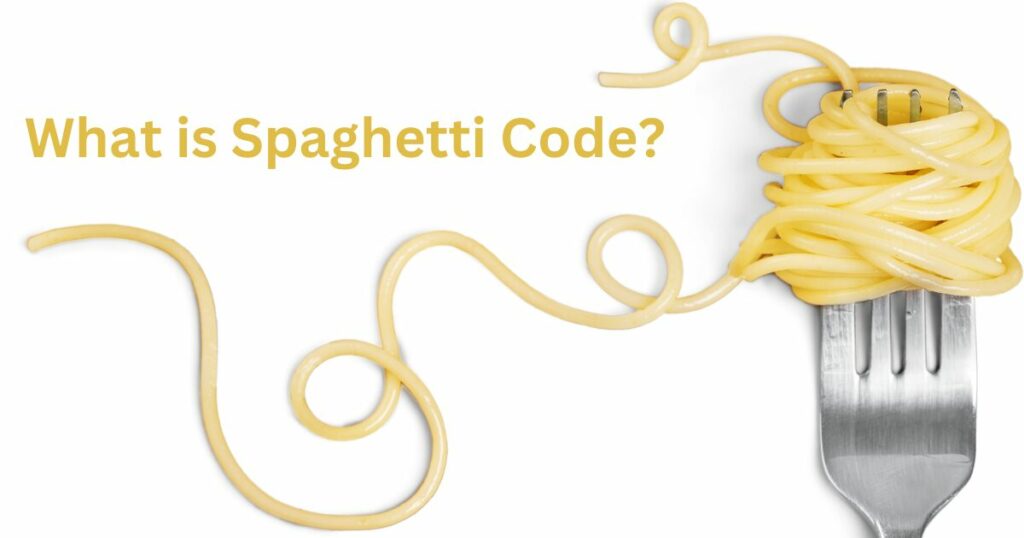
In programming, spaghetti code refers to complex and convoluted software with twisted logic –– It is challenging to read, understand, and maintain.
Personally, I define spaghetti code based on its readability. If I can’t easily follow the data flow from top to bottom or if my understanding of the code is incorrect — I consider it spaghetti code.
Consider a simple math problem: finding the number 4. I solve it in two ways:
// This resembles spaghetti code in programming:
Simple Solution:
2 + 2 = 4
Complex Solution:
1 + 7 – 12 + 6 + 6 – 4 = 4
The first solution is easy and tidy — It efficiently completes the job!
The second solution involves unnecessary steps and makes it harder to grasp its logic.
Historically, spaghetti code included excessive use of goto statements. Programming languages have evolved over the years, so explicit spaghetti code is less common today…
…but challenges still exist in code structure and organization!
While the classic examples of spaghetti code are less common today — the underlying principles remain relevant!
Medical Analogy
Imagine a hospital where you treat a patient on a bed with various tubes and support devices.
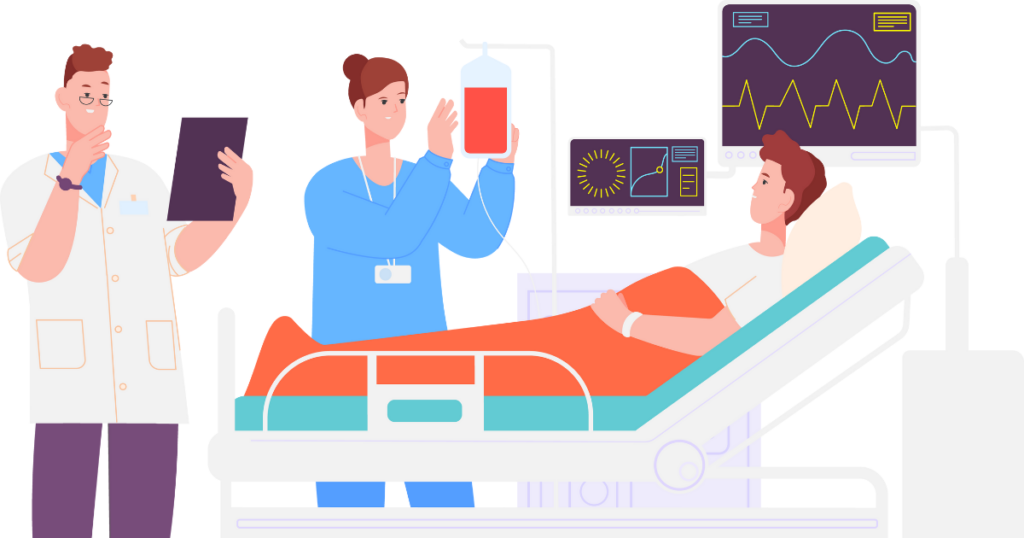
When a hospital first admits a patient, you configure a specific setup to meet their medical needs.
Once the treatment is complete, in an ideal world, you would reset the bed — remove the tubes, and clear everything — for the next patient with their requirements.
However, in IT, we rarely start afresh for each new “patient” (i.e., project or requirement).
Instead, we must manipulate the new “patient” (project or system) to fit into the configuration left by the previous one. When you need to replace tubing, you don’t remove it — you simply run the new tubing alongside the old…
Furthermore, the original staff (devs who set up the system) left the organization without documentation and tons of hand-written “DON’T REMOVE!!!!” warnings.
I love this analogy — it is simple and showcases how we create spaghetti code.
Basic Example in JavaScript
Good Code
for (let i = 0; i < 10; i++) { console.log(i); }
Bad Code
function printAllNumbers() { printFirstNumbers(); printMiddleNumbers(); printFinalNumbers(); } function printFirstNumbers() { console.log("1"); console.log("2"); console.log("3"); } function printMiddleNumbers() { console.log("4"); console.log("5"); console.log("6"); console.log("7"); } function printFinalNumbers() { console.log("8"); console.log("9"); console.log("10"); }
The “Good Code” uses a simple for loop, while the “Bad Code” breaks the task into multiple functions: printAllNumbers, printFirstNumbers, printMiddleNumbers, and printFinalNumbers.
The “Spaghetti” approach technically works, but it’s unnecessarily complex, hard to read, and violates the DRY principle — “Don’t Repeat Yourself!”
You might think, “Nobody would ever write code like the second example,” especially in a professional setting.
However, I’ve seen it all after years of web dev and teaching and assisting learners.
The reality — such code structures do exist in real-world production environments!
And if you think the Spaghetti Code is wild, wait until you see the ravioli code, or the macaroni code, or the lasagna code!
Main Causes of Spaghetti Code
Spaghetti code often involves global variables, changing throughout the code.
Suppose two functions, “functionA” and “functionB,” where B depends on A:
// Global variable let globalState = 0; function A() { globalState = 1; } function B() { if (globalState === 0) { console.log("Function A needs to be called first."); } else { console.log("Function B can proceed."); } }
globalState triggers specific behaviors in B (e.g., a negative value triggers an unusual action), and this is hard to understand and maintain.
If A is called before B 95% of the time, but the remaining 5% of the time it’s not — it creates an inconsistent code behavior.
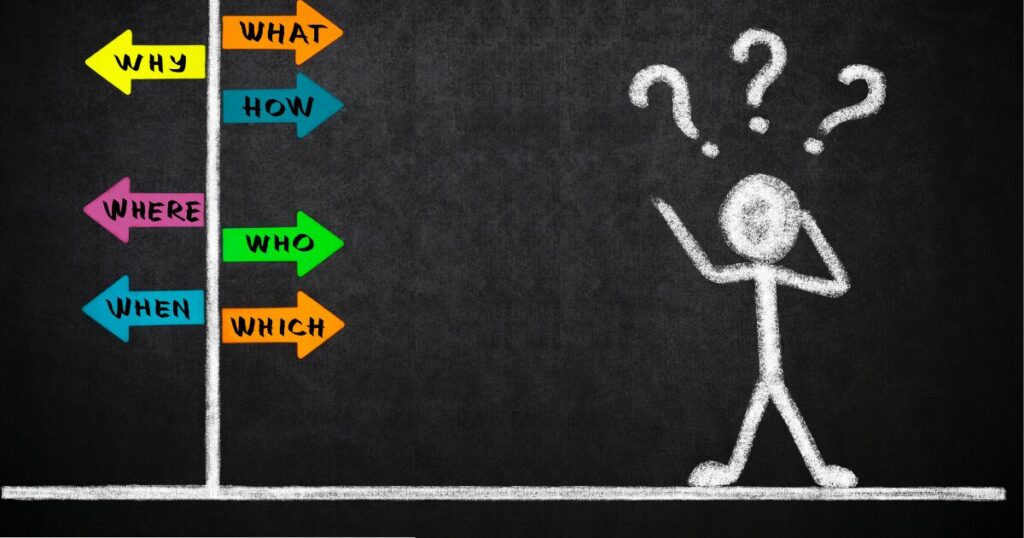
Impacts of Spaghetti Code on Development
Spaghetti code is like trying to follow a tangled web of noodles — it’s hard to make sense of what’s happening just by reading it.
While you follow the code to some extent — there’s a high chance of misunderstanding or missing something crucial!
It often violates the DRY (Don’t Repeat Yourself) principle — we repeat the same logic in multiple places, making the code harder!!
At its worst, changes in one part of the code unexpectedly affect seemingly unrelated parts…
…This leads to confusion, especially when a code is passed to a new development team.
Hard To Maintain And Debug
Maintenance refers to fixing or extending code YEARS after it was originally written!
When code is well-organized and clear, it’s easier to come along later and make updates or changes.
However, spaghetti-like code requires twice as much time untangling it (figuring out how it works) as maintaining it.
To debug spaghetti code, you need technical skills and patience…
…and a systematic approach! This leads to high development time and costs.
How I, As a Developer, Identify Spaghetti Code
I think it all comes down to coupling.
Coupling measures how closely a software system connects different classes, functions, or modules.
The types of coupling range from low (loose) to high (tight), with low coupling being the ideal state — due to its flexibility and ease of maintenance.
High coupling indicates a problematic code structure, where changes in one module necessitate changes in others.
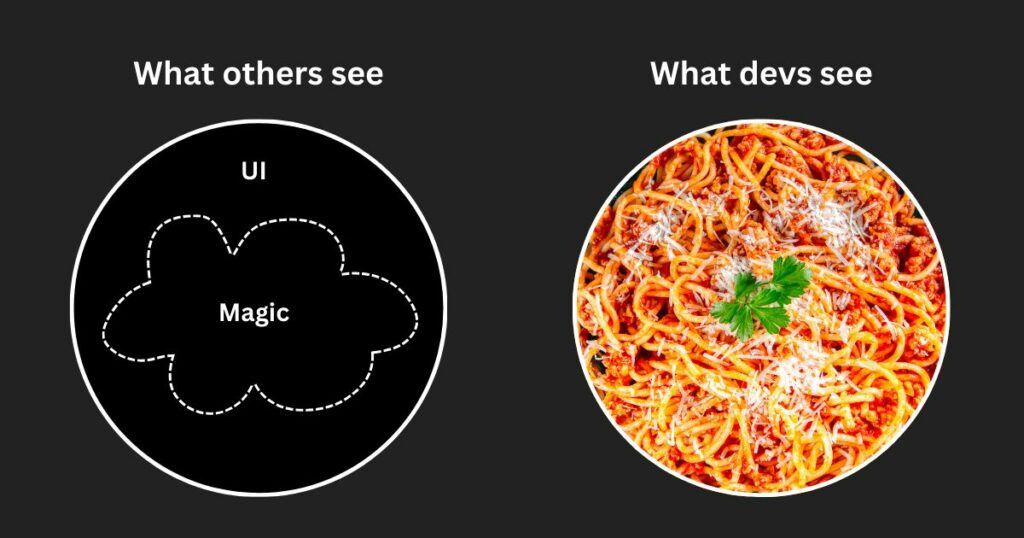
The more we couple things — the more rigid and brittle the system becomes!!
For example, if you change a function, and this update requires changes in several other functions (some of them surprisingly unexpected) — you have tightly coupled code =(
Spaghetti code often begins innocuously — a small workaround here, a quick fix there— but over time, it evolves into a complex mess challenging to understand and maintain.
As requirements evolve too, new features grow the codebase organically, often without a clear plan or structure.
Each addition, as well as each new developer, adds another layer to the dish.
As more developers work on the codebase — dancing around makeshift solutions — they add their own temporary fixes.
With time, the codebase angles into a mess of interdependencies, much like a plate of spaghetti with sauce and cheese.
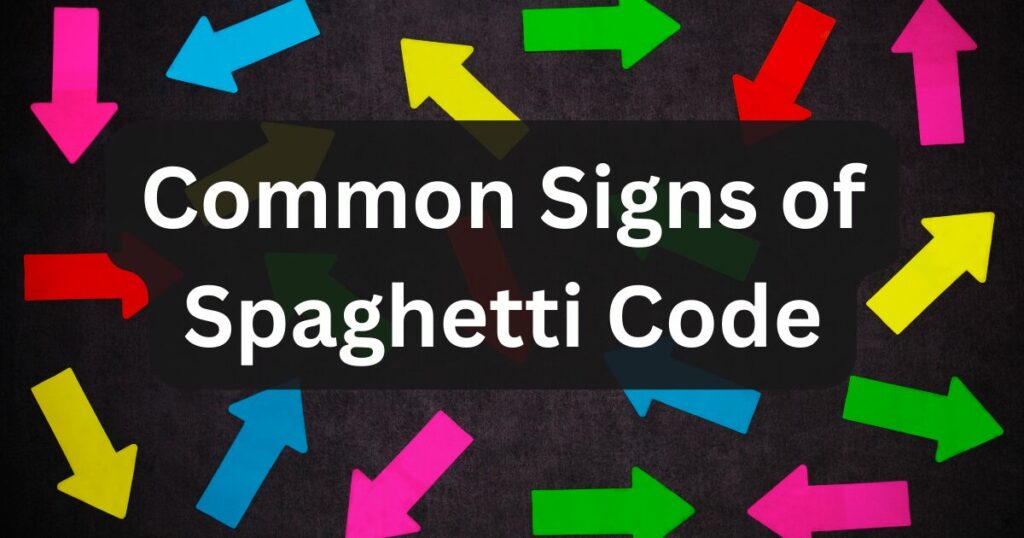
Common Signs of Spaghetti Code
Functions spanning hundreds of lines are difficult to understand at a glance.
It is hard to track deeply nested if-for-if-while structures and understand the logic at each level.
If I scroll up and down multiple files to follow a single function’s logic, I consider it spaghetti enough.
In my experience, hard-to-read code is almost always a sign of bad code! (regardless it functions correctly or not)
Part 3: What Is Not A Spaghetti Code
Not all poorly structured or repetitive code qualifies as spaghetti code.
A function that repeats the same block multiple times without breaking it into a function violates the DRY (Don’t Repeat Yourself), but it isn’t necessarily spaghetti code.
Overusing break or continue statements within loops leads to unstructured code but does NOT automatically result in bad code.
Spaghetti code is more than repetition — it includes complex, intertwined logic.
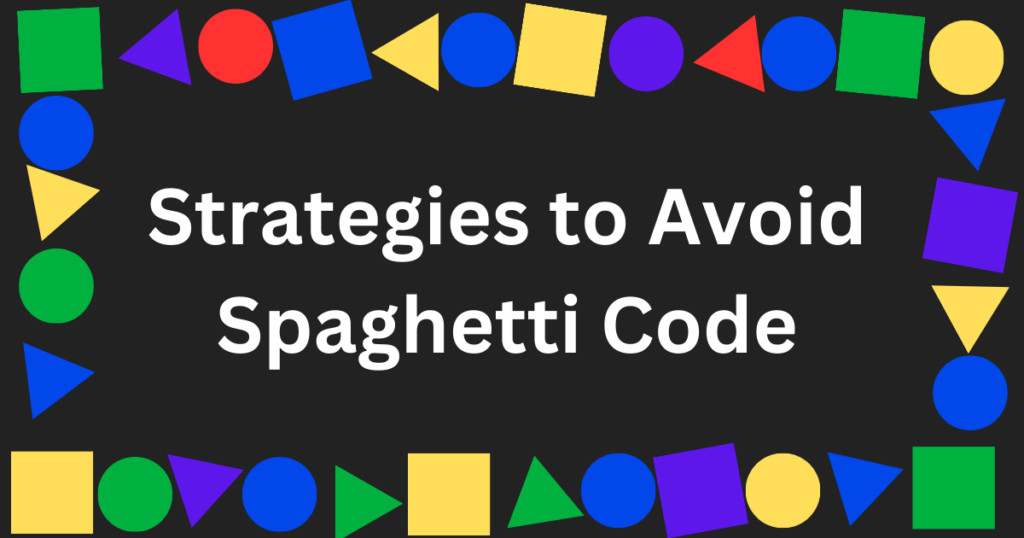
Strategies to Avoid Spaghetti Code
First, I recommend the “Clean Code” book for every programmer, regardless of experience level.
Robert Martin, known as Uncle Bob, covers everything from naming to error handling and OOP (object-oriented programming )!
But as a general rule — write small, focused functions that perform a specific task!! Ideally, these functions are around 10 lines long, tho this rarely happens to me, lol!
Break down your code into smaller, more manageable functions that read like a coherent narrative.
Some other necessary steps you can take:
- Use a version control system to track changes and collaborate with other devs.
- Integrate CI/CD automation.
- Report and track bugs in bag tracking systems.
- Using static code analysis tools!
- Maintain consistency in everything: naming, code structure, and design patterns.
- Increasing test coverage is a great idea in most vases!
Communicate
Communication is key!
Keep open and honest communication with project stakeholders, including business owners and team members.
It’s common to assign new devs to large projects with minimal guidance. This situation often results in “spaghetti code”.
Ask for help! Seek guidance, and remember that your first solutions will not be perfect! Learning from each project is more important than getting everything right first.
You must also explain your development process and your challenges in simple terms. I usually ask an experienced colleague to help with the technical details.
Training sessions or workshops work great to familiarize the team and yourself with the project!
Code Readability
Write clear, easy-to-understand code that flows logically from one section to the next and has obvious intentions.
The clearer the code, the simpler to identify and fix bugs!
IT companies highly value developers who write readable code because they produce less error-prone and more stable codebases.
Follow the principle of least surprise — your code should behave in a way that other developers expect it to — with no hidden functionalities or unexpected side effects!
Use comments to clarify not apparent elements.
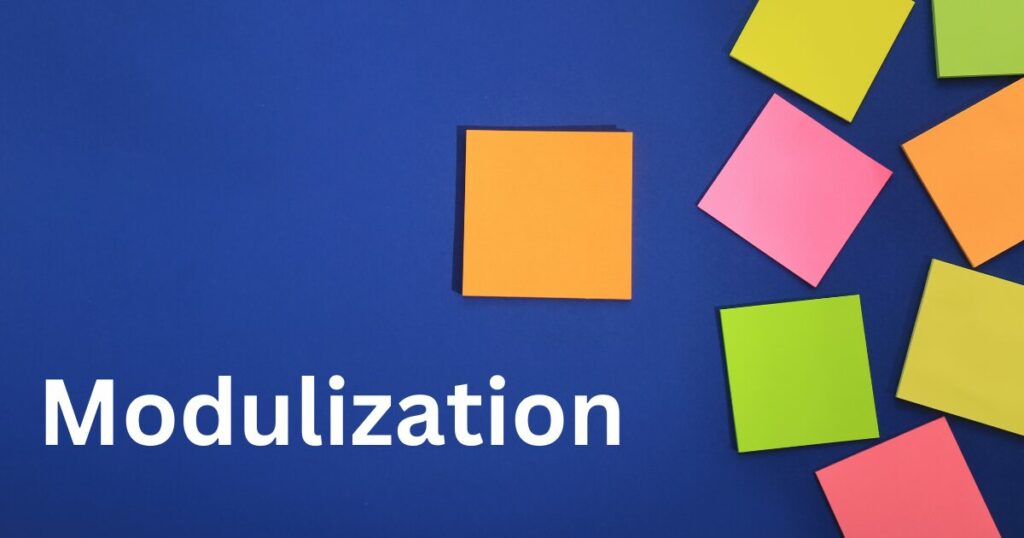
Modulization
Modularization is a strategic approach to breaking down a large codebase into independent segments — modules.
Identify areas that can be isolated into modules. Your first modules might not be small or perfectly independent initially. However, even partial modularization simplifies the code.
Separate these areas from the main body of the code. Refine each module — break down large functions into more specific ones or classes.
With modularization, you focus on one module and interface at a time! Refactor without the pressure of addressing the entire codebase simultaneously.
Code Architecture and Structured Programming
Code architecture is essential for creating maintainable and scalable software that other devs can easily understand.
Thoughtfully organize and structure code components! Standard structures across projects reduce the learning curve.
Consistent code structures help developers quickly locate components and understand project architecture. Otherwise, you’ll start from scratch each time!!
Clarify complex logic and interactions within the code with flow chart equivalents (graphical representations or text-based descriptions).
Minimize the use of global variables — only when necessary!
As Uncle Bob says, organize methods within a class based on the degree of abstraction:
- High-Level Abstract Methods at the top of your class.
- Concrete Implementation Methods should be lower in the class.
Maintainable Code Documentation
In software development, code and documentation are inseparable. Documentation helps you understand and work with the code, regardless of when you join the project.
Just as a novel guides the reader through its narrative, well-structured code docs guide you through the project logic.
Integrate a single source of truth (Document Management System — DMS) for all project-related information. Follow standard rules and conventions across all documentation.
Update project docs regularly — include links to relevant commits, issues, and builds!
Version Control Systems Commit Logs
Like a good wine, the value of commit logs increases over time.
It’s common to undervalue commit logs in source control management (SCM), often leaving only a cryptic one-line description for significant changes.
Always include a reference to the task or issue ID related to the commit.
Your commit log is a history record of changes to a project’s codebase — you and other developers will review it months or years from now. Keep it clear enough to explain the reasons and context of the changes made.
Rule of Thumb: When writing a commit log, ask yourself, “Will my log provide all the information if I search for details about this change in the future?” If the answer is no — update the comment.
Code Comments
Code comments serve as an in-line guide for developers! They help to explain the purpose and logic of the underlying code.
Adopt the “Explain Like I’m Five” (ELI5) approach to commenting. Break down your explanations as simple as possible.
Focus on each line and practice describing what a line of code does rather than commenting on whole sections or functions.
This especially helps me when I revisit my own code after some time.
Comments can become outdated as code evolves — update them as you modify your project!
Keep comments constructive and avoid unnecessary noise or drama. We are professional developers, tho it’s common for us to express emotions through comments!
Unit Testing And TDD
TDD is a practice in which developers write tests before the code itself. This methodology helps continuously test the codebase.
Legacy code often lacks tests, making it risky to change. When dealing with such code:
- Introduce unit tests to any new changes!
- Increase test coverage percent and depth of tests — start with critical paths and features.
Familiarize yourself with automated testing tools and frameworks as they save tons of time.
Also, actively try to break your code to find bugs — your users will do the same.
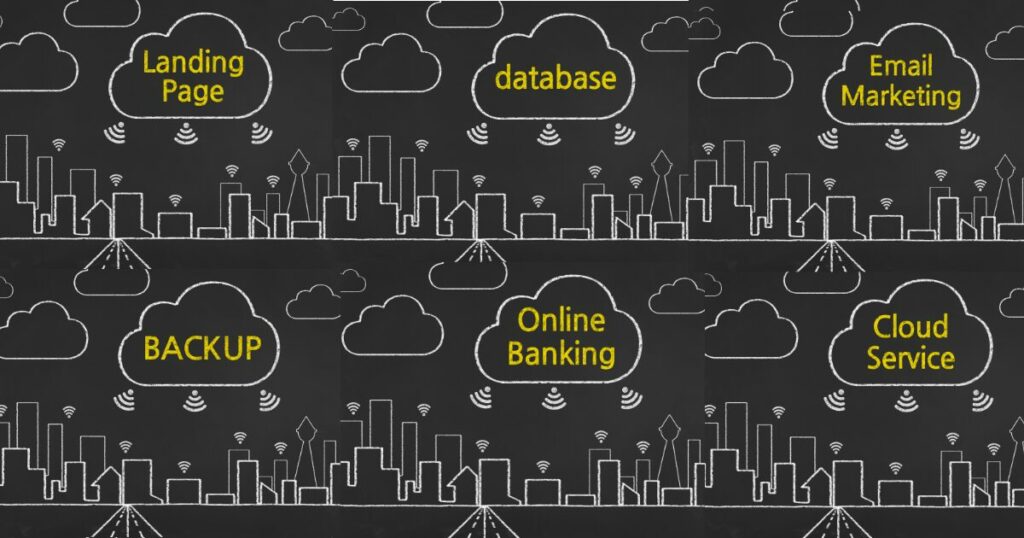
Common Mistakes Leading to Spaghetti Code
Lack of planning often results in rapid prototyping without a long-term structure.
Each new feature leads to a tangled codebase! =( As a result, coders spend more time maintaining code than writing new features.
These are some other common mistakes that lead to spaghetti code:
- No modularity — all code within a single file or function.
- Poor organization code with unclear functionality and purpose.
- Long functions that handle multiple tasks.
- Poor naming and non-descriptive names.
- Global state modification that alters the global state without clear docs.
- Insufficient documentation and lack of comments.
- Lack of code reuse.
- Lack of testing.
Time Pressure
In the fast-paced IT world, programmers face significant pressure to meet tight deadlines. As a result, the elegance and efficiency of the code become secondary considerations.
Under time constraints, developers resort to quick fixes and workarounds rather than addressing the root of issues.
As more devs contribute, the codebase grows in complexity and reverse engineering consumes a significant amount of time trying to figure out what the code does…
…and why it was written that way. Plus, diverse programming styles vary among developers and add additional challenges!
Code Duplication and Lack of Modularization
Code duplication and a lack of modularization lead to a cluttered codebase!
Duplicate code leads to larger codebases — you need to duplicate any update or bug fix from one in other sections.
Each module should have a single responsibility! In object-oriented code, isolate operations such as validating data, merging data layers, and saving data into separate methods or classes.
Methods that require numerous parameters are confusing and error-prone. As well as long functions that extend beyond a screen length!
Spaghetti Code Refactoring
Imagine working on a project where you have a 5,000+ lines long file, each packed with numerous function calls and no tests.
Start by comprehending the major functions and their roles within the file.
Ensure you understand the code’s functionality before making changes.
Begin with a small update that simplifies the existing code. Write docs and comments, or start discussions with original devs (if possible).
Note that refactoring, especially in large systems, carries the risk of breaking something that previously worked.
Code Refactoring – Worth It?
Refactoring of the code that you do not well understand can break parts of the code that previously worked.
In legacy safety systems, even if the code is outdated, the priority is reliability and proven functionality.
Also, the financial and practical implications outweigh the perceived benefits. For example, if the code is functional and stable, management prioritizes maintaining the status quo over investing in refactoring…sight…
Refactoring is not a one-size-fits-all solution. Make the decision based on the code impact, risks, and long-term benefits.
Refactoring vs. Full Rewrite
Starting from scratch with a full rewrite is always tempting, but it pauses development on existing products and halts business operations. This can leave the business without a product and delay feature development.
Gradually migrating (refactoring) the existing codebase towards a cleaner architecture allows you to improve the code without disrupting its functioning.
Fix Legacy Project Code Smell
A systematic approach minimizes risk throughout the process!
1. Establish a testing foundation and focus on adding tests before making any changes — document the existing functionality first!
2. Make small (mechanical) changes that do not alter code behavior — extract repeated blocks into separate functions.
3. Start clarifying smaller functions — rename variables or simplify expressions.
4. Making more significant changes that impact the overall architecture.
Simplicity is Key! Ensure that each change is simple enough for reviewers to verify that behavior is preserved. The simpler the change, the easier it is to review and accept.
If high-risk changes meet resistance, focus on low-risk improvements, progressing the project toward better code quality.
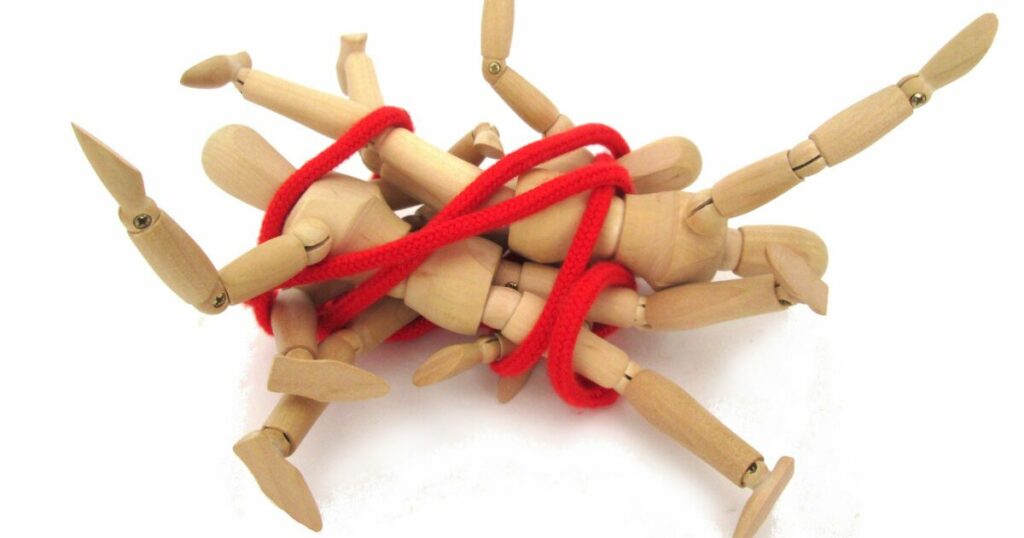
Conclusion:
Spaghetti code hinders the progress and quality of any software project!
The fight against spaghetti code is ongoing, but we can win with the right tools and attitudes!
Cultivate a culture of clean coding within your team, and watch your projects thrive in a chaos-free environment.